Javascript Click Handlers
Javascript Files
When using the default theme, the /themes/default/resources/js/theme.js file is loaded with the default calendar page. Any javascript code that exists in this file will be loaded and available as well. When writing javascript click handlers, this is a good place to write your click handler functions as they will be loaded when your calendar loads.
If you are using a custom theme, you will need to ensure your function is loaded when the calendar page loads.
/*****************************************************************************************
* Namespace setup
* This creates the namespaces for functions used in this appication.
* This only needs to be done once.
*
****************************************************************************************/
if (!KD.example) {
// create the KD.example namespace if it doesn't already exist
KD.example = {};
}
if (!KD.example.handler) {
// create the KD.example.handler namespace if it doesn't already exist
KD.example.handler = {};
}
/*****************************************************************************************
** Custom Click Handler functions
****************************************************************************************/
/**
* ShowEvent click handler
* This click handler is fairly simple as it just displays the event object's property
* names and values.
*
* @param calEvent - the clicked event object (not the element on the page, but the actual event)
*/
KD.example.handler.ShowEvent = function ( calEvent ) {
alert(YAHOO.lang.dump(calEvent));
}
Results of executing the ShowEvent click handler:
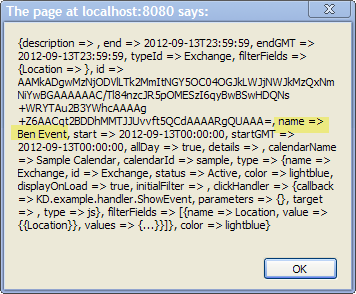
Admittedly this example doesn't do much other than to dump the event details into a javascript alert window, but it serves to illustrate how a javascript click handler can be defined, and what information is available. For instance, looking at the image above, you can see the event name property can be accessed in the javascript function by accessing the event variable's name property. Since the event variable in this function is called calEvent, this looks like calEvent.name, or calEvent['name']. All of the other event properties can be accessed in the same way.
Available Event Properties
For a list of available Event properties that can be accessed from the event object, please see Event Properties.
Updated over 2 years ago