Building a Bridge Adapter
What you need to know to connect your Kinetic Platform to the world
What is a Bridge Adapter?
A Bridge Adapter is a java class that is deployed to and managed by a Kinetic Agent. Normally a Bridge Adapter is used to make a request into a system to retrieve data. A Bridge Plugin is a configured instance of a Bridge Adapter. The Bridge Adapter's functionality is exposed through three implementation methods count, retrieve and search; more information on the methods can be found below.
Why build your own Bridge Adapter?
You might be asking yourself, "if Kinetic Data already provides so many integrations, why would I need to build my own?" The answer is that there are so many systems to integrate with the hard-working folks at Kinetic Data couldn't possibly build them all. Because we know that we can't make everything, we provide you with a means to develop and deploy your Bridge Adapters.
Who can deploy a Bridge Adapter
If you are a fully on-prem customer or a customer who has deployed your own agent, you can deploy custom Bridge Adapters.
Kinetic Data employees build Bridge Adapters and deploy them to the System Agent.
What do I need to build a Bridge Adapter?
Your organization has access to an Agent, and you've decided you need a custom integration. What do you do now?
What is needed to build a Bridge Adapter:
- Java 8 Software Development Kit
- An IDE (NetBeans is a standard open-source IDE)
- A Java package manager (all Kinetic Data Bridge Adapter use Apache Maven)
- The Kinetic Agent Adapter (contact Kinetic Data to get a copy)
Once you have installed all the requirements, you can begin building a Bridge Adapter.
Bridge Adapter high-level overview.
The "looking out the airplane window" view of a Bridge Adapter is relatively simple. The Kinetic Platform and the Agent have a "special" connection that allows them to speak securely. The Agent receives requests from the Kinetic Platform and forwards them to the appropriate Bridge Adapter. The Bridge Adapter gets the request and takes action specific to that request. Usually, the Bridge Adapter will call out to a source system to fetch data. When the source system responds, the Bridge Adapter transforms the data and returns it to the Kinetic Platform.
Bridge Adapter anatomy
This is a reference section that can be skipped the first time through
- Bridge Adapters are Java classes that implement the BridgeAdapter class.
- Every adapter will likely require some configuration. Kinetic Data exposes a ConfigurableProperty class used to build the properties.
- Bridge Adapters have methods to Override:
- getName() returns the name of the adapter used by the platform UI.
- getVersion() returns the version of the adapter.
- getProperties() returns the configurable properties and their current values for the current bridge adapter.
-setProperties(parameters) sets the configurable properties to the values supplied in the passed parameter map - initialize() is commonly used to make a connection to the source system.
- This method is called after the bridge has been created for the first time and then any time the properties are updated after that.
- count(bridgeRequest) implementation method to get a count of the structure.
- retrieve(bridgeRequest) implementation method to get a single result of the structure.
- search(bridgeRequest) implementation method to get a multiple results of the structures.
Not all methods must be implemented
There are times when a method does not make sense to implement. The source system called by the adapter may not support counting the number of records. In this case, the adapter must still define the method, but it is common to return a BridgeError from the method.
- The Bridge Adapter's implementation methods count, retrieve, and search have specific return types. The Kinetic Agent Adapter exposes the classes used for return types.
- count must return a Count class.
- retrieve must return a Record class.
- search must return a RecordList class.
- A RecordList is made up of Record objects.
The BridgeRequest class
Using a Model, the platform generates a request to send to the Agent. The Agent transforms the request into a BridgeRequest and provides the methods count, retrieve, and search the BridgeRequest as a parameter.
Fields on the BridgeRequest class:
- fields is a list defined by the Model.
- To define a new field, go to Attributes > Mapping of the Model. Hit the $ in the field.
- metadata currently, this can only be populated using a Javascript generated request.
- parameters is a list defined by the Model.
- To define a new parameter go to Qualifications > Query of the Model. Hit the $ in the field.
- parameters are defined in the Query field of the Model, but passed as a separate object in the request.
- query is the query string defined by the Model.
- The query is "raw" in the sense that it has not done a replacement of the parameters.
- structure is a string defined by the Model.
- The intent of a Structure is to capture the schema of the Model.
The Fields on the BridgeRequest class have getters:
- getFields
- getMetadata
- getParameters
- getQuery
- getStructure
A deep dive into the BridgeRequest
Each of the implementation methods (count, retrieve, search) uses the BridgeRequest object for processing a request. A BridgeRequest object is created from the REST API call to the Kinetic Agent. The agent receives and translated an incoming request an provides the implementation method with a BridgeRequest that has the following fields:
Structure
The type of data that is being searched for (ie. Submissions, Users, Incidents, etc.). Similar to a Table in a database.
Fields
A list of fields that will be returned for each record that is found and returned from the data source.
Query
A query that will be used to search the records that match the given structure in the data source. Any records that match the query will be returned from the bridge.
Metadata
A map of the metadata that was passed to the bridge to refine the search of the data source. The most common key-value metadata pairs passed are Order and Pagination (pageNumber, pageSize, offset OR pageToken), but any key-value pair can be passed as metadata and used in the bridge. This is the best place to pass through any data that you need to search the data source but doesn't fit under the Structure, Fields, or Query categories.
Parameters
A map of parameter names and values that will be used to substitute out parameter placeholders for actual values in the query before a call to the data source is made.
Important files/components
Qualification Parser
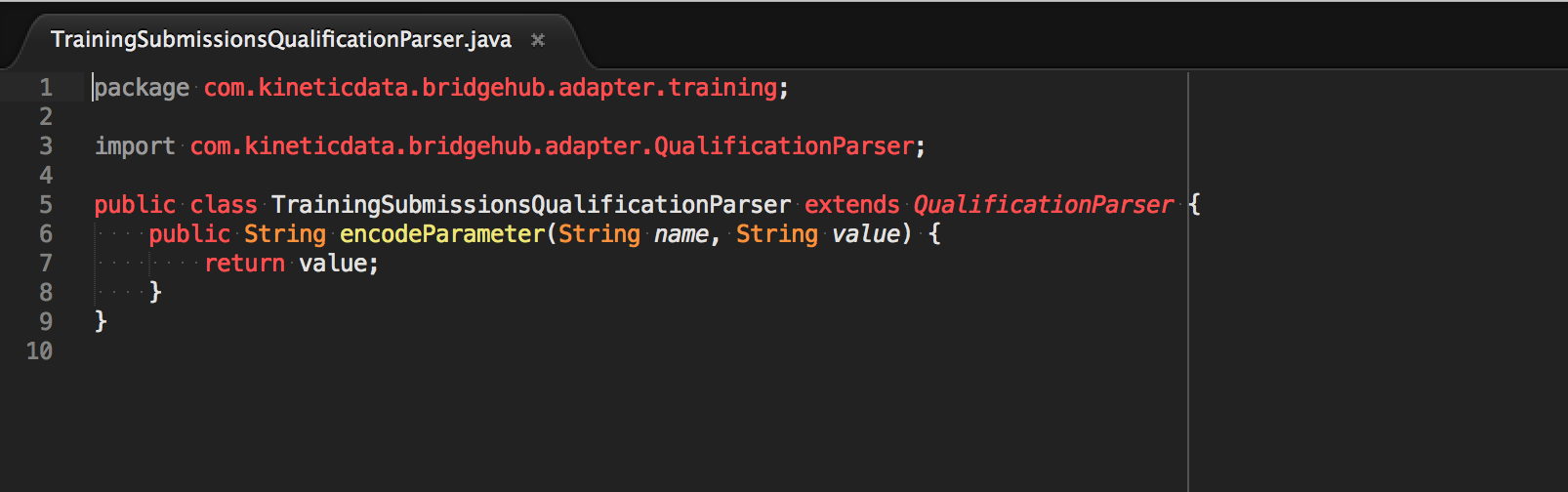
The qualification parser is a class created to help parse and encode parameters for bridge qualifications. The only method that is required to be implemented in the Qualification Parser is the abstract method encodeParameter. The method is passed a name and value for a parameter and the method needs to know how to encode it so that the parameter value conforms to the standards of the data source before it is attempted to be sent as a part of the query. The most common place where this is used is for data sources that use XML and the encodeParameter method is used to escape reserved XML characters so the adapter doesn't send malformed XML to the data source.
Discovery File
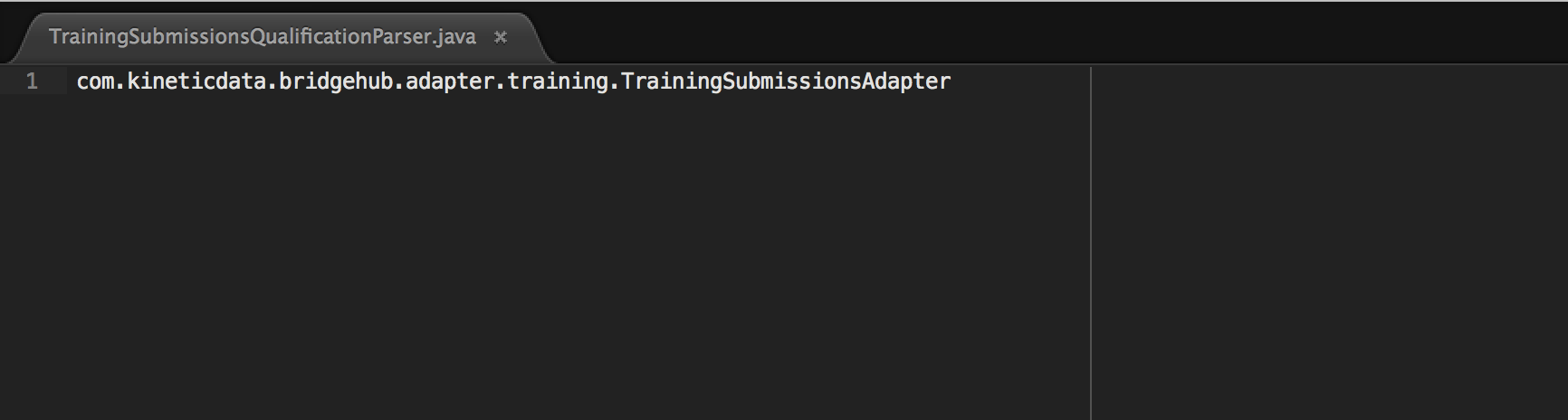
In a netbeans project the discovery file is located at src/main/resource/META-INF/adapters. It needs to be a file named com.kineticdata.bridgehub.adapter.BridgeAdapter that contains the class for your main adapter file. The Kinetic Agent searches through all .jar files in the kinetic-agent/WEB-INF/lib for any that have the discovery file. The agent then attempts to load up the Bridge Adapter if the discovery file was found.
Example: The Kinetic Core adapter's discovery file contains the following:
com.kineticdata.bridgehub.adapter.kineticcore.KineticCoreAdapter
jUnit Test File (Optional)
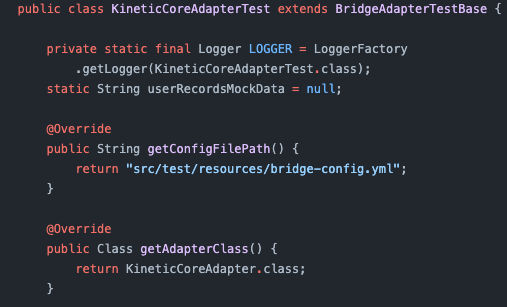
Snippet of the Kinetic Data Platform Bridge Adapter jUnit file.
A jUnit test file can be included to allow testing an adapters functionality without loading it into a Kinetic Agent. This isn't required, but it allows you to test situations that have a static set of inputs and expected outputs much (ie. Testing that bad inputs lead to the correct errors).
Updated over 2 years ago