Building a Bridge Adapter
What you need to know to connect your Kinetic Platform to the world
What is a bridge adapter?
A bridge adapter is a .jar file that is deployed to and managed by a Kinetic Agent. In most cases, a bridge adapter is used to make a request into a system to retrieve data. A bridge plugin is a configured instance of a bridge adapter. Bridge adapter functionality is exposed through the count
, retrieve
, and search
implementation methods. We'll discuss these methods more later in this topic.
Bridge adapters give you a "looking out the airplane window" view. The Kinetic Platform and the Agent have a connection that allows them to speak securely. The Agent receives requests from the Kinetic Platform and forwards them to the appropriate bridge adapter. The bridge adapter gets the request and takes action specific to that request. Usually, the bridge adapter will call out to a source system to fetch data. When the source system responds, the bridge adapter transforms the data and returns it to the Kinetic Platform.
Why build your own bridge adapter?
You might be asking yourself, "If Kinetic Data already provides so many integrations, why would I need to build my own?" The answer is that there are so many systems to integrate with, we couldn't possibly build them all. Because we know that we can't make everything, we provide you with a means to develop and deploy your bridge adapters.
Who can deploy a bridge adapter?
If you use a customer-managed or have deployed your own agent, you can deploy custom bridge adapters.
What do I need to build a bridge adapter?
Let's say your organization has access to an Agent, and you've decided you need a custom integration. What do you do now?
To build a bridge adapter, you will need:
- The Kinetic Agent Adapter
- A Java 8 Software Development Kit (SDK)
- An Integrated Development Environment (IDE), such as the open-source IDE NetBeans.
- A Java package manager. All Kinetic Data bridge adapters use Apache Maven, but you can use whichever package manager you prefer.
Once you have installed all the requirements, you can begin building a bridge adapter.
Bridge Adapter Anatomy
The bridge adapter .jar file includes multiple Java classes, which are explained in detail below. Every adapter will likely require some configuration. Kinetic Data exposes a ConfigurableProperty
class used to build the properties.
Bridge adapters include the following properties:
- Structures: The type of data being searched for (for example, submissions, users, or incidents). The structure is similar to a table in a database.
- Fields: A list of fields for each record that is found and returned from the data source.
- Query: Used to search records that match the given structure in the data source. Any records that match the query are returned from the bridge.
- Metadata: A map passed to the bridge to refine the data source search. Any key-value pair can be passed as metadata and used in the bridge. The most common pairs passed are Order and Pagination (for example,
pageNumber
,pageSize
,offset OR pageToken
). Metadata is the best place to pass through any data needed to search the data source that doesn't fit in the Structure or Query categories.Note: Metadata can only be set by calling a bridged resource directly or by using the bridgedResources JavaScript helper function.
- Parameters: A map of names and values used to substitute parameter placeholders for actual query values before the data source is called.
Override Methods
Bridge adapters require the following override methods:
Method | Purpose |
---|---|
getName() | Returns the name of the adapter used by the platform UI. |
getVersion() | Returns the version of the adapter. |
getProperties() | Returns the configurable properties and their current values for the current bridge adapter.-setProperties(parameters) Sets the configurable properties to the values supplied in the passed parameter map. |
initialize() | Commonly used to make a connection to the source system. This method is called after the bridge has been created for the first time and then any time the properties are updated after that. |
count(bridgeRequest) | Gets a count of the structure. Must return a Count class. |
retrieve(bridgeRequest) | Gets a single result of the structure. Must return a Record class. |
search(bridgeRequest) | Gets multiple results of the structures. Must return a RecordList class (which is made up of Record objects.). |
Note: The count, retrieve, and search implementation methods have specific return types. The Kinetic Agent Adapter exposes the classes used for return types.
It's not required for every method to be implemented. In some cases, it might not make sense to implement a method. For example, the source system called by the adapter may not support counting the number of records, but overriding the method is still required. As such, the adapter must still define the method, but the method will most likely return a BridgeError.
The count
, retrieve
, and search
methods use the BridgeRequest object for processing a request. A BridgeRequest object is created from the REST API call to the Kinetic Agent. The agent receives and translates the incoming request and provides the implementation method with a BridgeRequest that includes the BridgeRequest classes described below.
The BridgeRequest class
The Platform generates requests to send to the Agent using Models. The Agent transforms the request into a BridgeRequest and provides the count
, retrieve
, and search
methods to the BridgeRequest as a parameter.
The BridgeRequest classes use the following fields:
- Fields: A list defined by the Model. New fields are defined in the Mapping field of the Attributes tab. Uses the
getFields
getter. - Metadata: The Model metadata. Currently, this can only be populated using a JavaScript-generated request. Uses the
getMetadata
getter. - Parameters Aa list defined by the Model. New parameters are defined in the Query field of the Qualifications tab but are passed as a separate object in the request. Uses the
getParameters
getter. - Query The query string defined by the Model. The query is "raw" in the sense that the parameters are not replaced with values. Uses the
getQuery
getter. - Structure A string defined by the Model used to capture the schema of the Model. Uses the
getStructure
getter.
Qualification Parser
The qualification parser is a class created to help parse and encode parameters for bridge qualifications. It only requires implementation of the the encodeParameter
abstract method. The qualification parser method is passed a name and value for a parameter and needs to know how to encode it so that the parameter value conforms to the standards of the data source before the query attempts to send it. In most cases, the qualification parser is used for data sources that use XML. The encodeParameter
method is used to escape reserved XML characters so the adapter doesn't send malformed XML to the data source.
Discovery File
The discovery file must be named "com.kineticdata.bridgehub.adapter.BridgeAdapter" and must contain the class for your main adapter file. The Kinetic Agent searches through all .jar files in the Kinetic-Agent/WEB-INF/Lib folder to locate the discovery file. If the discovery file is found, the agent attempts to load the bridge adapter.
Example: The Kinetic Core adapter's discovery file contains the following class:
"com.kineticdata.bridgehub.adapter.kineticcore.KineticCoreAdapter"
jUnit Test File (Optional)
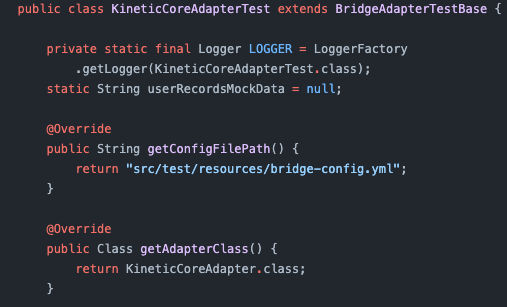
Snippet of the Kinetic Data Platform Bridge Adapter jUnit file.
You can include a jUnit test file in the .jar file to test an adapter's functionality without loading it into a Kinetic Agent. While not required, it allows you to test situations that have a static set of inputs and expected outputs. For example, you might include a series of bad inputs to test whether those bad inputs lead to the correct errors.
Updated 11 months ago