How to Validate Fields Before Submitting a Form
The standard field validation validates a field when the form is submitted, but it is possible to extend this capability to the blur event for the field (as soon as the user leaves the field). This is done by leveraging the K('field['+fieldName+']').validate()
function and setting up an event for the appropriate field on page load using the jQuery blur function.
In this example, we will validate an IP address field and display an error if the field pattern doesn't validate when the user leaves the field or submits the form.
First, we'll set up the "IP Address" field to validate the length of the user entry using a regex pattern.
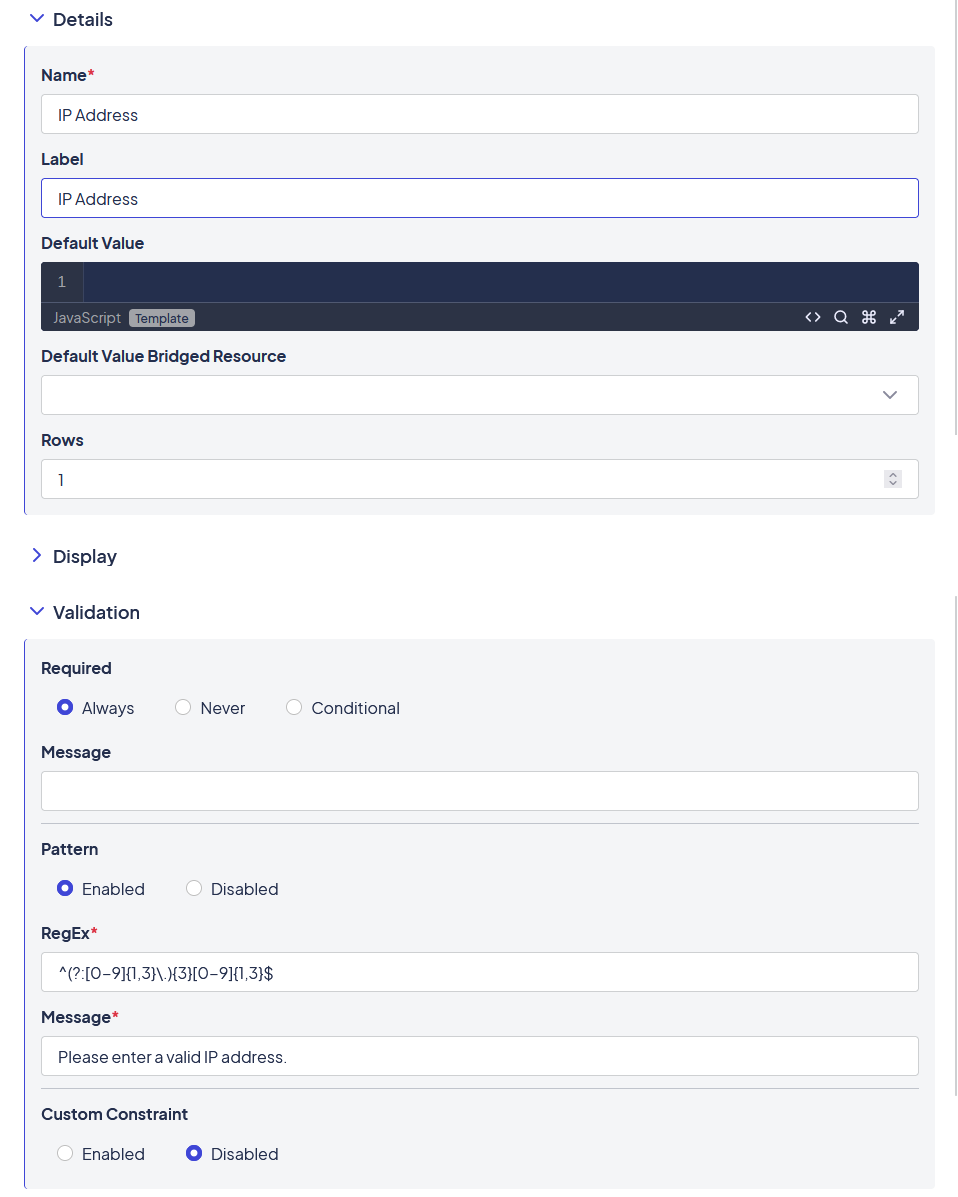
Next, we'll add a load event to validate that the value entered in the IP address field matches the regex pattern (and blur if it does not). This calls the validation function for the field with that class and displays the error messages for any errors it finds.
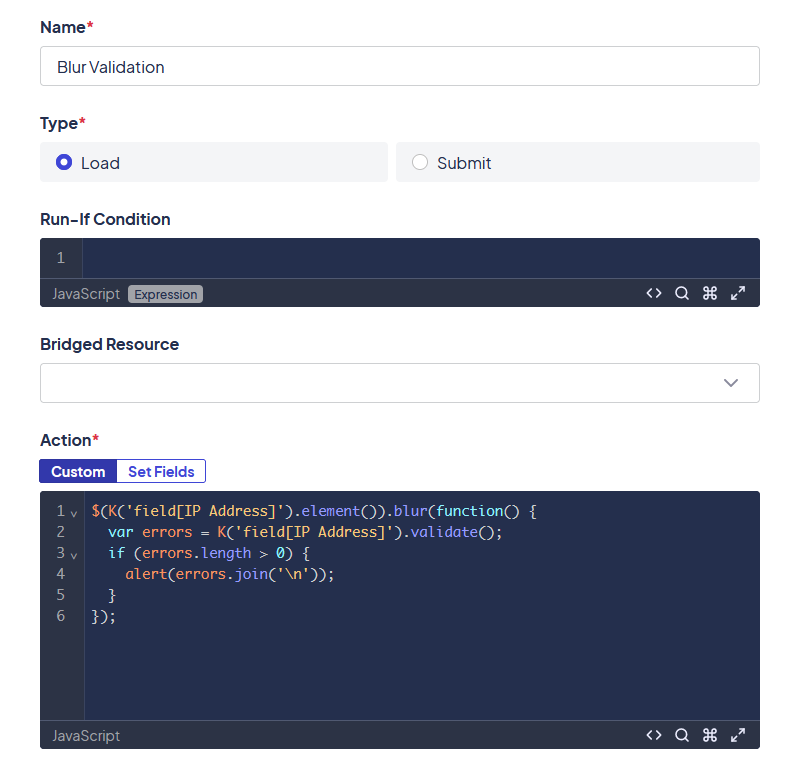
$(K('field[IP Address]').element()).blur(function() {
var errors = K('field[IP Address]').validate();
if (errors.length > 0) {
alert(errors.join('\n'));
}
});
Updated 11 months ago