How to Disable Specific Checkbox or Radio Button Options in a Form
Sometimes, you may want to show users a radio button or checkbox question but not allow them to choose from all the options. This solution describes how to use jQuery to accomplish that.
Usage
Specific radio buttons or checkbox options within a list question can be enabled/disabled on the fly (in response to the user's selection/entry into another field). This is done in two steps:
- Create a function that uses Kinetic functions to enable/disable the radio buttons and place the function in the Custom Header Content of the service item.
- Call this function with an event
Example
This example uses a question called Store to limit/change what is available in a following question called Spices. The front end looks like this:

- There is a script UpdateChoices that updates the 'disabled' attribute for the individual inputs (radio buttons) for the list question you want to modify. This function is put in the Custom Header Content in the Form Settings of the item.
<script>
function UpdateChoices(store) {
//get the value for the question question for spices
//so we know if we need to null it out later (because it
//becomes an invalid choice)
qstnValue = K('field[Spices]').value();
//enable/disable the radio button options by updating the
//'disabled' attribute for that particular input value for the
//qstn id found above
if (store == "Supermarket") {
//Enable Dill because we can get that at the supermarket
$(K('field[Spices]').element()).filter('[value=Dill]').attr('disabled',false);
//Disable Elderflower because we can't get that at the supermarket
$(K('field[Spices]').element()).filter('[value=Elderflower]').attr('disabled',true);
//Note that black pepper and salt doesn't need to be mentioned
//because it's enabled for all three options
//Clear the selection if the now disabled item(s) where chosen
if (qstnValue == "Elderflower")
K('field[Spices]').value(null);
} else if (store == "Butcher") {
//Disable Dill and Elderflower because we can't get that at the butcher
//Note that black pepper and salt don't need to be mentioned
//because they are enabled for all three options
$(K('field[Spices]').element()).filter('[value=Dill]').attr('disabled',true);
$(K('field[Spices]').element()).filter('[value=Elderflower]').attr('disabled',true);
//Clear the selection if the now disabled item(s) where chosen
if (qstnValue == "Elderflower" || qstnValue == "Dill")
K('field[Spices]').value(null);
} else {
//Enable Dill and Elderflower because we can get them at the herbalist
//Note that black pepper and salt don't need to be mentioned
//because they are enabled for all three options
$(K('field[Spices]').element()).filter('[value=Dill]').attr('disabled',false);
$(K('field[Spices]').element()).filter('[value=Elderflower]').attr('disabled',false);
}
}
</script>
Now add that to the header for the service item.
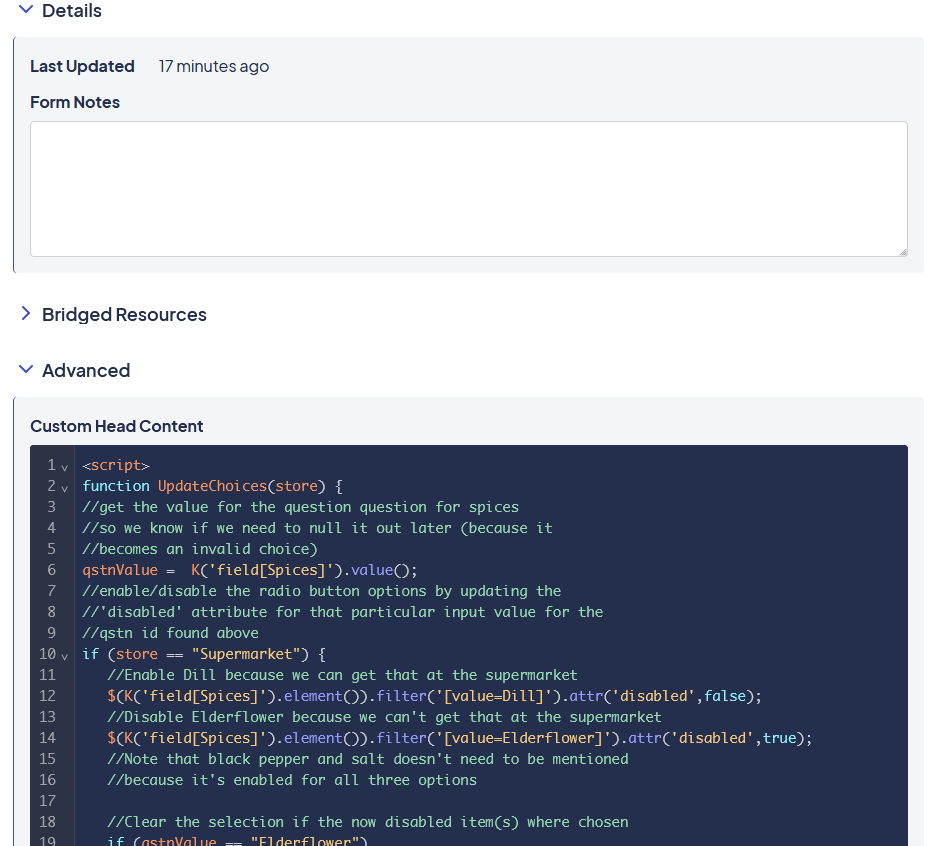
- Now create an event on the Store question that calls the UpdateChoices function on change.
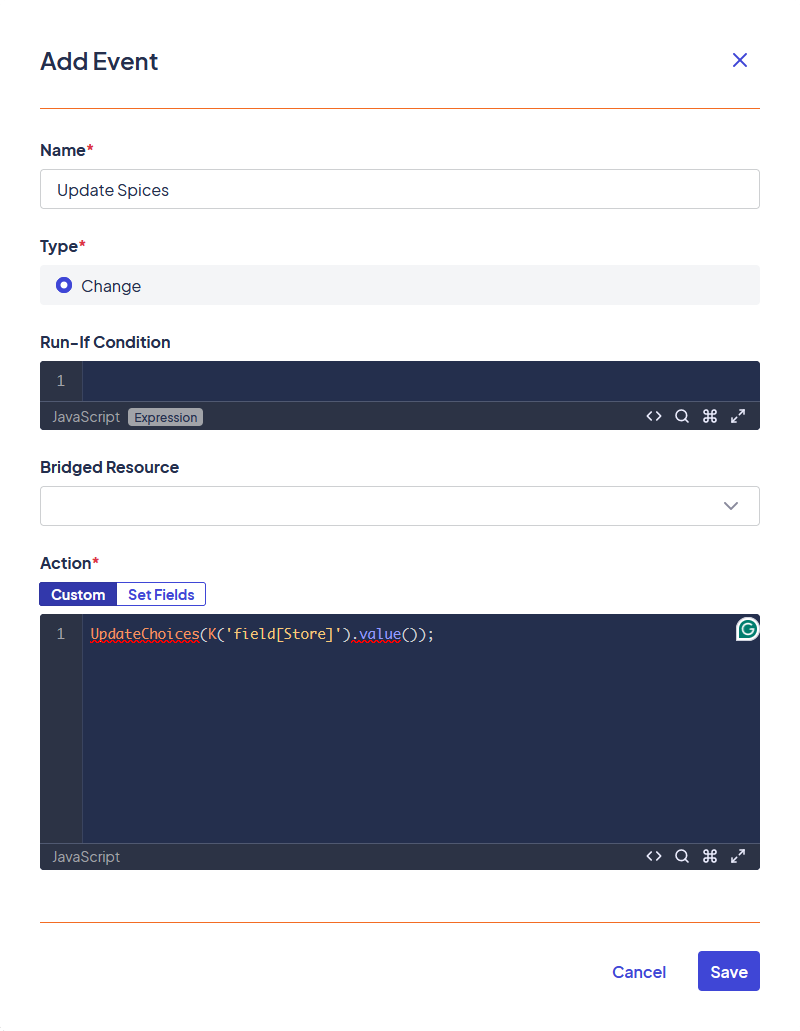
Updated 10 months ago