How to Use Bridges with JavaScript
Sometimes, you may want to control a bridge action in greater detail than setting fields, filling in field defaults, or leveraging the DataViewer library to build tables. For example, you may need to chain several bridge calls to get your final result. Or perhaps you need to do special processing on the result once it's returned. This requires you to use JavaScript to work with the bridges.
First, you'll need to create a bridged resource on the form. Once you've created the resource, you can access it with a load method that takes an options object as an argument. This object has the following properties:
Property | Purpose |
---|---|
attributes | An array of attributes that you want to return. |
metadata | A map of metadata parameters that will be passed to the bridge adapter. The supported metadata parameter names depend on the bridge adapter used by the active bridge mapping. |
values | A map of values referenced by your bridged resource parameters. |
success | This function is called when the bridged resource request is complete. Takes one argument, the bridged data. |
error | This function is called when an error is encountered while processing the bridged resource request. It takes one argument: the error response from the server. |
Here is an example of a bridged resource load:
// Load a bridged resource named 'People' that has no parameters:
K('bridgedResource[People]').load({
attributes: ['First Name', 'Last Name', 'Login Id'],
metadata: {
"limit": "5",
"pageToken": "i0j70ogl9638s4ccs0bvdkrqftxa6kvzt52",
"order": "Login Id:asc"
},
success: function(bridgedData) {
// Do something with the bridged data response.
// For example:
console.log(bridgedData.records);
}
});
// Load a bridged resource named 'Person' that has a parameter. The
// parameter is configured to reference the value of the Login ID field,
// which is defined in the Form Builder:
K('bridgedResource[Person]').load({
attributes: ['First Name', 'Last Name'],
values: {'Login ID' : 'Allen'},
success: function(bridgedData) {
console.log(bridgedData.record);
}
});
In addition to the load
method, you can call these other methods:
Method | Purpose |
---|---|
K('bridgedResource[People]').name() | Returns the name of the bridged resource. |
K('bridgedResource[People]').type() | Returns the bridged resource type (either “Single” or “Multiple”). |
K('bridgedResource[People]').dependencies() | Returns an array of field names that are used in parameters. |
K('bridgedResource[People]').attributes() | Returns an array of values that will be returned from the bridge. |
K('bridgedResource[People]').metadata() | Returns a map of metadata properties sent to the bridge. |
Example
As with any use of bridges, you must set up a model with qualifications and attributes. In this case, we are using a model mapped to the Kinetic Platform user object.
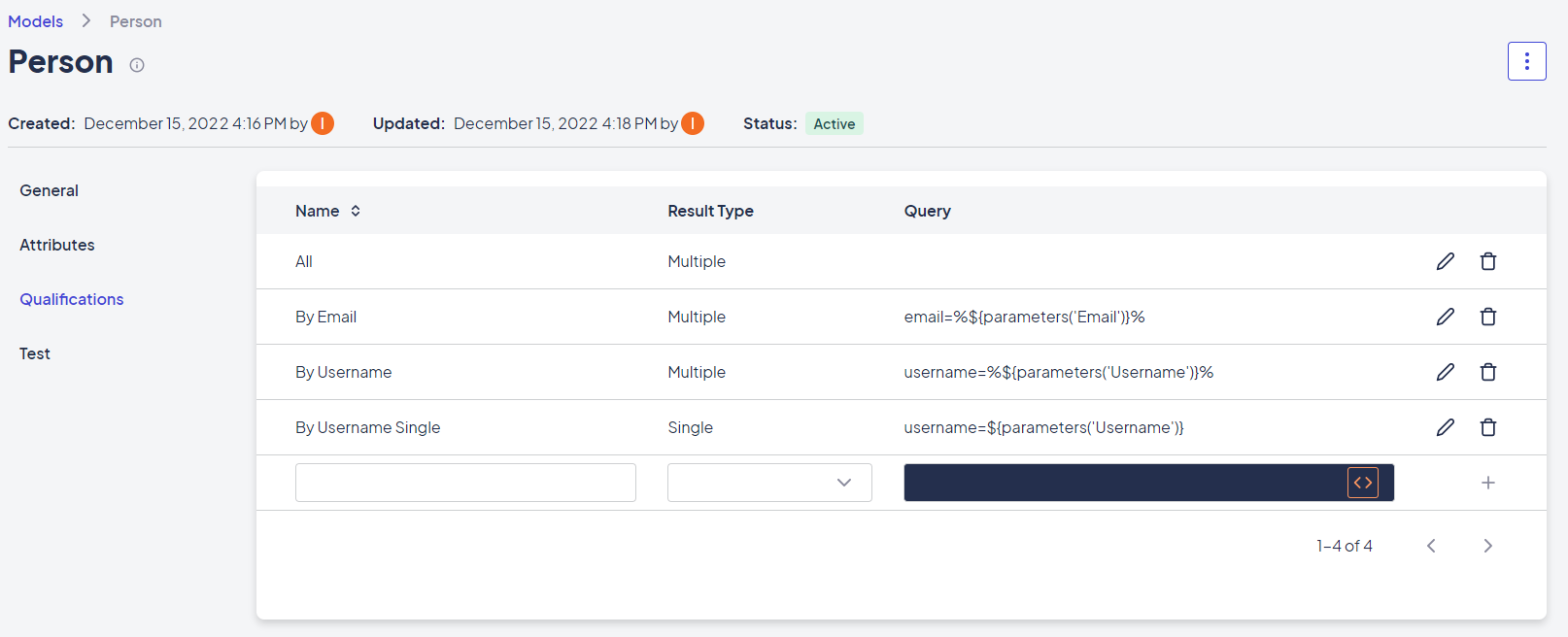
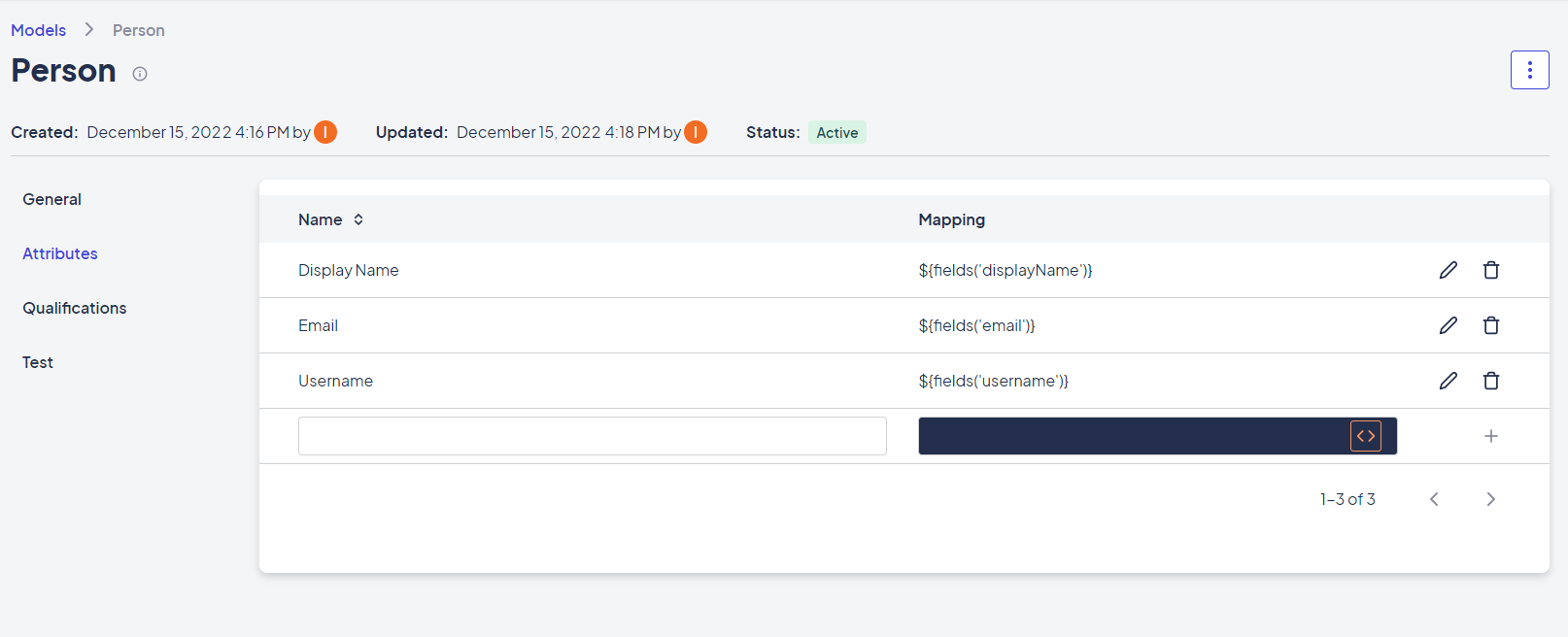
Then the bridge resource needs to be set up in the service item.
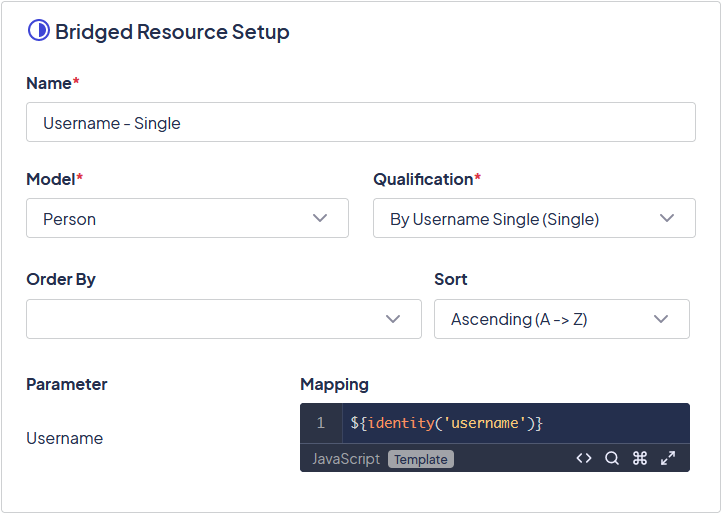
In this case, the bridge is being used within an on-load event:
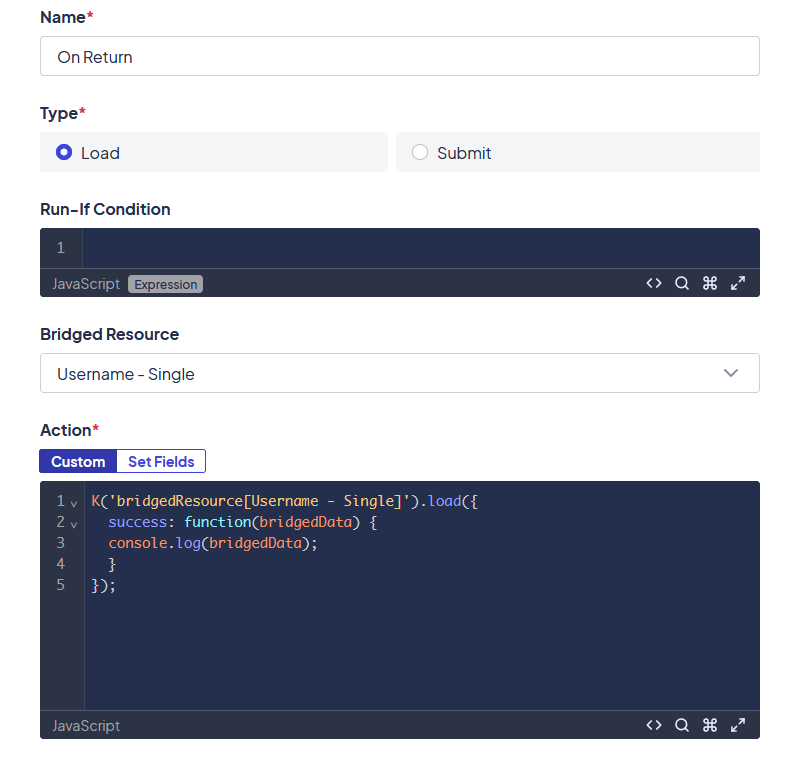
This particular example logs the result into the browser's console without specifying any attributes or parameters. The bridged resource takes care of the parameter, and all of the attributes will be returned.
Working with Returned Results
How you can work with your results will vary slightly based on whether the bridge you call is a single or multiple result type qualification. Single result type qualifications return an object of the attributes returned, such as:
{Display Name: "Anne Ramey", Email: "[email protected]", Username: "[email protected]"}
Multiple result type qualifications will return an array of objects even if there is only one object returned, such as:
[{Display Name: "Anne Ramey", Email: "[email protected]", Username: "[email protected]"}]
Note: It is more likely that a single result type query will lead to an error on no match rather than an empty string. This depends on the system being searched and they query being used, but it is something to be cautious of.
The most common way to work with these bridge results is to parse them as JSON and work with the JSON objects/arrays.
Chaining Bridge Calls
Bridge calls are asynchronous. If you want to be sure that one bridge call is happening upon returning on another, you must chain them together. This can be done in a couple of ways. First, you can use the first bridge call to populate/change a field with the data needed for the second bridge call, which would run on the change of that field. This is the cleanest and most direct way. If that doesn't fit the scenario for some reason, you can embed one bridge call within the success function of the other.
Updated 6 months ago